React Typescript [Vite]
npm create vite@latest
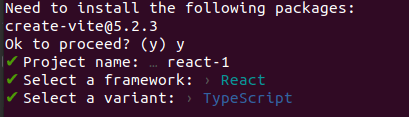
npm install --save-dev eslint prettier eslint-config-prettier eslint-plugin-prettier @typescript-eslint/parser @typescript-eslint/eslint-plugin husky lint-staged @commitlint/{cli,config-conventional} eslint-plugin-import-helpers
Development Dependencies for React with Typescript
--save-dev: Saves dependencies only for the development environment.
eslint: Tool to analyze and find problems in JavaScript and TypeScript code.
prettier: Code formatter that ensures a consistent style throughout the project.
eslint-config-prettier: Disables ESLint rules that might interfere with Prettier.
eslint-plugin-prettier: Integrates Prettier with ESLint to show formatting errors.
@typescript-eslint/parser: Parser that allows ESLint to understand TypeScript.
@typescript-eslint/eslint-plugin: Set of specific TypeScript rules for ESLint.
husky: Tool to create Git hooks, helping automate tasks before committing.
lint-staged: Runs linters on staged files in Git.
@commitlint/cli: Command-line tool to ensure commit messages follow a conventional standard.
@commitlint/config-conventional: Configuration for commitlint to follow the conventional commit message format.
eslint-plugin-import-helpers: ESLint plugin to automatically order imports.
Eslint Config
.eslintrc.js
module.exports = {
root: true,
env: { browser: true, es2020: true },
extends: [
'eslint:recommended',
'plugin:@typescript-eslint/recommended',
'plugin:react-hooks/recommended',
'prettier',
],
ignorePatterns: ['dist', '.eslintrc.js'],
parser: '@typescript-eslint/parser',
plugins: ['react-refresh', 'prettier' , 'eslint-plugin-import-helpers'],
rules: {
'react-refresh/only-export-components': [
'warn',
{ allowConstantExport: true },
],
'prettier/prettier': ['error'],
'react/react-in-jsx-scope': 'off',
"react/prop-types": "off",
'no-multiple-empty-lines': ['error', { max: 1, maxEOF: 1 }],
"import-helpers/order-imports": [
"warn",
{
newlinesBetween: "always",
groups: [
["/^next/", "module"],
"/@styles/",
"/@components/",
"/@lib/",
["parent", "sibling", "index"],
],
alphabetize: { order: "asc", ignoreCase: true },
},
],
},
}
Prettier Config
prettier.config.js
module.exports = {
"useTabs": false,
"singleQuote": false,
};
Commitlint Config
commitlint.config.js
module.exports = { extends: ["@commitlint/config-conventional"] };
Husky
npx husky install
pre-commit
#!/bin/sh
. "$(dirname "$0")/_/husky.sh"
npx lint-staged
commit-msg
#!/usr/bin/env sh
. "$(dirname -- "$0")/_/husky.sh"
npx --no -- commitlint --edit
Package.json
type: module
type: commonjs
package.json
"format": "prettier --write .",
"lint": "eslint --fix . --ext .js,.jsx,.ts,.tsx",
"prepare": "husky install"
Lint Staged Config
.lintstagedrc
{
"**/*.{js,jsx,ts,tsx}": ["npm run format", "npm run lint"]
}
Ignore Eslint
.eslintignore
dist
package-lock.json
husky
configs
env
public
server.js
jsconfig.json
package.json
public
README.md
.eslintrc
.eslintrc.json
.eslintrc.js
.prettierrc.json
.eslint*
commitlint.config.js
next-env.d.ts
postcss.config.js
prettier.config.js
Ignore Prettier
.prettierignore
dist
package-lock.json
configs
env
husky
public
server.js
jsconfig.json
package.json
public
README.md
.eslintrc
.eslintrc.json
.eslintrc.js
.prettierrc.json
commitlint.config.js
next-env.d.ts
postcss.config.js
commitlint.config.js
prettier.config.js